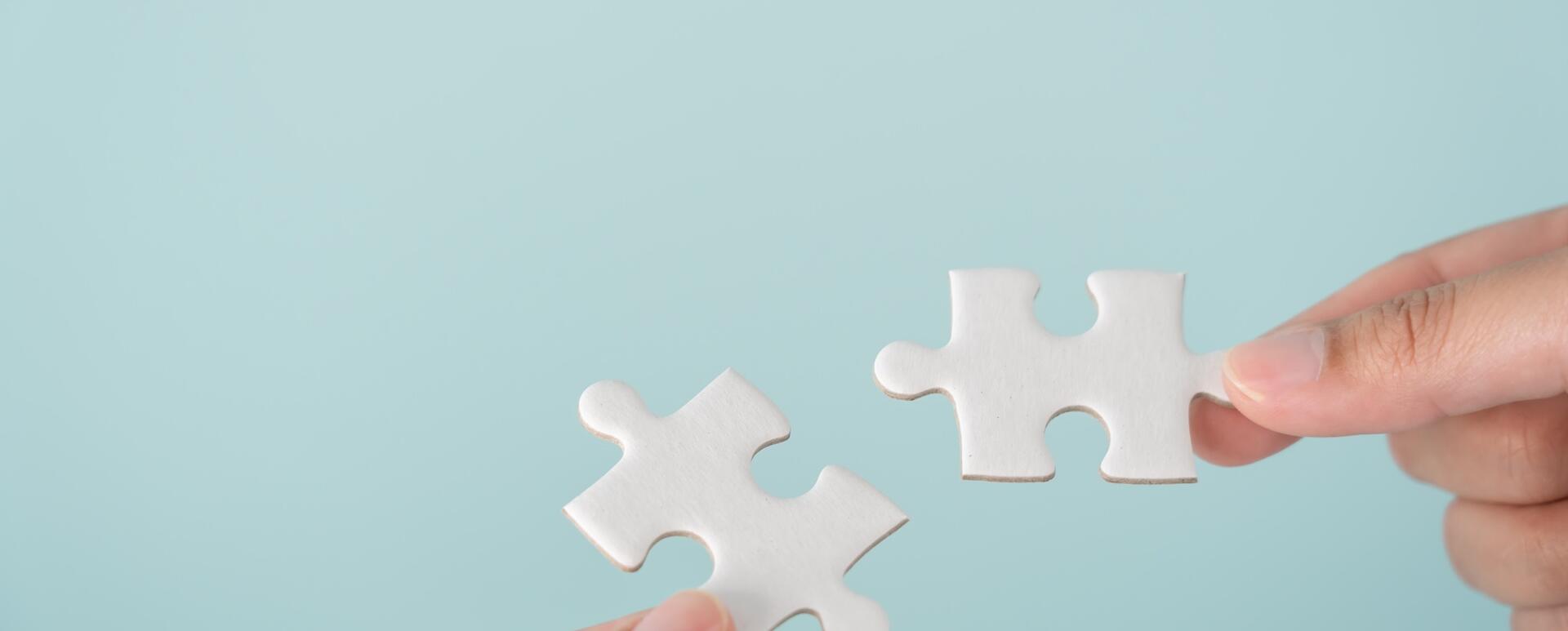
How to Perform a Drupal Integration with Other Enterprise Systems?
Effective integration of different systems in a company today is an elementary factor for success. In a world of dynamic technologies and IT tools, Drupal stands out because it’s a constantly evolving open source software with the support of a large community. With its flexible structure of modules written in PHP and the ability to use tools such as Composer, Drupal becomes an ideal solution for integrating various programs in an organization.
Why does Drupal work well for integration?
In favor of Drupal is its flexibility and the rich database of modules available on Drupal.org, which is constantly growing and often includes ready-to-use solutions for integration.
It’s also worth noting that Drupal has its API, which makes it much easier to create custom Drupal integration modules. This popular system is based on Symfony, which allows for writing new integrations even faster and easier, thanks to access to advanced, ready-made solutions. You don't have to start everything from scratch, which saves time and resources.
In our blog post, we'll discover how to harness Drupal's potential to effectively integrate it with other systems in your organization, taking advantage of the powerful tools and instant options available in this powerful open source software.
How is Drupal architecture built?
Architecture plays a crucial role in the context of Drupal integration with other systems. This is especially true in a dynamic business environment, where a company's systems are often subject to modification and must be easily extensible and ready for change.
As technologies and business requirements evolve, Drupal's flexible design enables rapid implementation of changes and the addition of new features and integrations. This is critical to maintaining competitiveness and operational efficiency in the enterprise.
PHP language
Drupal is written in PHP – a well-known language used worldwide by web developers. How does its popularity affect Drupal integrations with other systems?
The PHP language is widely used in the development of web applications, resulting in unique toolkits for programmers known as SDKs (Software Development Kits). Examples include the SDK for Google services or the SDK for eBay integration.
Ready-made libraries, numerous tutorials, and documentation on system connection in PHP are also beneficial. Thus, the process of Drupal integration with other systems becomes much more accessible and efficient.
Symfony components
Drupal and Symfony allow you to install off-the-shelf libraries using the Composer tool, which further simplifies the process of integrating with external programs. These libraries are often provided by the systems you want to integrate with, which means that companies can use official or unofficial solutions from manufacturers.
As a result, the integration process becomes even smoother, and ready-made libraries make it easier to create connections and exchange data between different platforms. This, in turn, speeds up the implementation of integration.
An example is the installation of Google services such as Google Drive or YouTube:
{
"require": {
"google/apiclient": "^2.15.0"
},
"scripts": {
"pre-autoload-dump": "google-autoload-dump::cleanup".
},
"extra": {
"google/apiclient-services": [
"Drive,
"YouTube"
]
}
}
Also noteworthy is the SDK provided by Amazon:
composer require aws/aws-sdk-php
Twig template system
Drupal uses the Twig template system to render content on websites. While it may seem unrelated to integration, it’s essential for Drupal's flexibility in this context. With Twig, advanced output content processing is possible, making it easier to communicate between different applications.
In addition, the Twig template system works with the library system in Drupal. It allows external JavaScript libraries, which expands the possibilities for creating user interfaces and customizing them for a given project. This way, Drupal becomes a more flexible integration tool, allowing us to create advanced web solutions.
For example, we can create custom services for formatting and filtering the displayed content. Here is an example of turning www.xxxx.xxx addresses into clickable links "on the fly":
service.yml
my_module_twig_extension.twig.InlinerTwigExtension:
class: drupal_module_twig_extension\TwigExtension\TwigExtension
Tags: { name: twig.extension }
Class code:
public function getFilters(): array {
return [
new TwigFilter('replaceUrls', [$this, 'replaceUrls']),
];
}
/**
* Replace url link to html link in texts.
*/
public function replaceUrls($text): array|string|null {
if (empty($text)) {
return $text;
}
$pattern = '/(http:\/\S+|https:\S+|www.\S+)/i';
$replacement = '<a href="$1" target="_blank">$1</a>';
$text = preg_replace($pattern, $replacement, $text);
$pattern = '/<a href="www.(.*?)">(.*?)</a>/i';
$replacement = '<a href="http://www.$1">$2</a>';
return preg_replace($pattern, $replacement, $text);
}
And in Twig, we add our custom filter:
{{text|replaceUrls}}
How to perform Drupal integration with other systems?
We’ve already reminded you of the basic elements of Drupal's architecture that can affect the system's connection with external tools. Below, we present specific approaches to integration.
Integration using Drupal Rest API
Integration with Drupal Rest API is a relatively simple and effective process. To be able to implement it, we need to enable the Rest module in the Drupal admin panel, configure the appropriate access permissions, and create API endpoints.
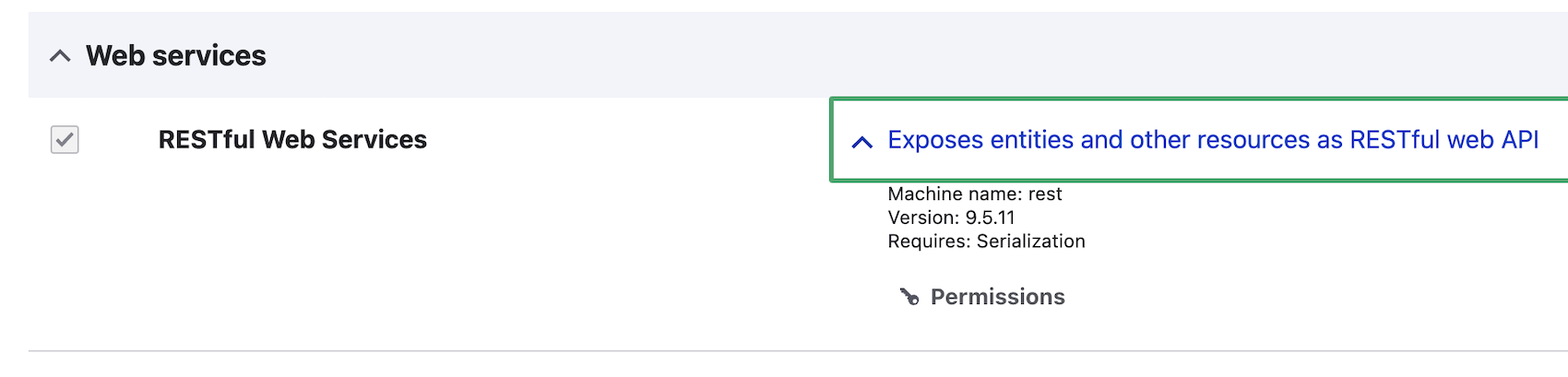
We can also use the REST UI add-on, which allows us to add some actions from the UI.
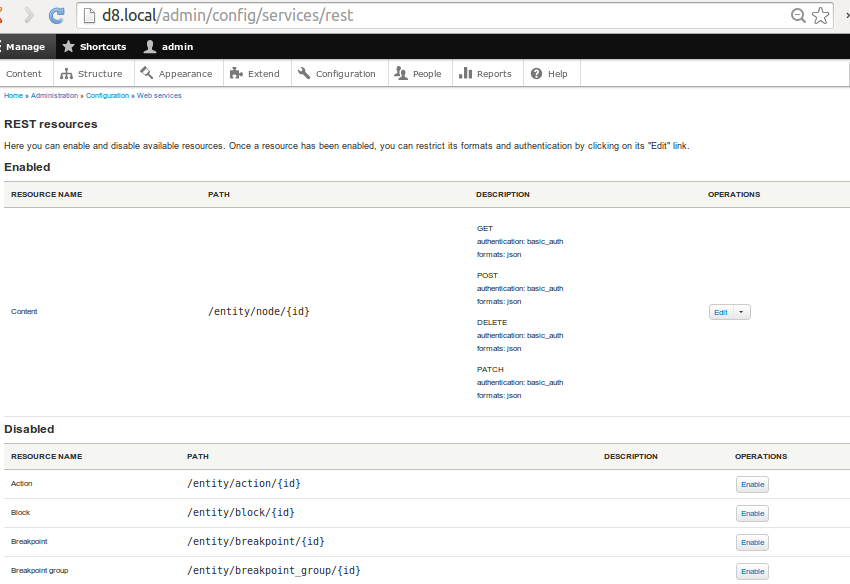
It’s also important to implement authorization and authentication mechanisms to ensure that API access is secure.
Drupal and Symfony allow the custom creation of REST endpoints. Developers can use tools such as Guzzle and other libraries to create custom API endpoints that meet the project's exact needs. This means that the structure and behavior of the API can be tailored to specific business requirements. As a result, we manage to build personalized integration solutions and gain accessible data exchange between Drupal or Symfony and other applications and platforms.
Before implementing an integration, it’s a good idea to thoroughly test the API and create documentation to make it easier for other developers to use the resources and operations provided by the API. This way, Drupal can be effectively integrated with other systems, allowing data and processes to flow freely between different applications and tools.
Creating a plugin for Rest
We need to create a classe with annotation @RestResource along with url_paths and extend RestResource class. Example:
<?php
Namespace Drupal_your_module_plugin_resource;
use Drupal;
use DrupalResourceResponse;
use SymfonyComponentDependencyInjectionContainerInterface;
use SymfonyComponentHttpKernelExceptionAccessDeniedHttpException;
/**
* Provides a resource to get view modes by entity and bundle.
*
* @RestResource(
* id = "my_custom_resource",
* label = @Translation("My Custom Resource"),
* uri_paths = {
* "canonical" = "/my-custom-endpoint".
* }
* )
*/
class MyCustomResource extends ResourceBase {
/**
* {@inheritdoc}
*/
public static function create(ContainerInterface $container, array $configuration, $plugin_id, $plugin_definition) {
return new static(
$configuration,
$plugin_id,
$plugin_definition,
$container->get('entity.manager')
);
}
/**
* Responds to GET requests.
*
* @return @DrupalResourceResponse.
* The HTTP response object.
*
* @throws @SymfonyComponentHttpKernelException.
* Throws exception expected.
*/
public function get() {
// Your custom logic here to handle GET requests.
// For example, you can fetch data and return it as a JSON response.
$data = ['message' => 'This is a custom REST resource.'];
return new ResourceResponse($data);
}
/**
* Responds to POST requests.
*
* @param mixed $data
* The data received in the POST request.
*
* @return @DrupalResourceResponse.
* The HTTP response object.
*
* @throws @SymfonyComponentHttpKernelException.
* Throws exception expected.
*/
public function post($data) {
// Your custom logic here to handle POST requests.
// For example, you can create a new resource based on the received data.
// Return a response indicating success or failure.
return new ResourceResponse(['message' => 'POST request handled.']);
}
}
Another approach is to create routing and controllers to receive our requests:
my_module.resource_list:
path: '/api/resources'
defaults:
_controller: '_controller::getList'.
_title: 'Get list of resources'.
methods: [GET].
requirements:
_permission: 'access content'
formats: ['json']
my_module.resource_get:
path: '/api/resources/{id}'
defaults:
_controller: '_controller::getSingle'.
_title: 'Get a single resource'
methods: [GET].
requirements:
_permission: 'access content'
options:
parameters:
id:
type: 'integer'
formats: ['json']
my_module.resource_create:
path: '/api/resources'
defaults:
_controller: '_drupal_module_Controller_ResourceController::create'.
_title: 'Create a new resource'
methods: [POST].
requirements:
_permission: 'create new resource'
options:
parameters:
date:
type: 'entity:my_resource'
formats: ['json']
In addition, we present how to download sample data in our custom module from external systems, such as YouTube, using GuzzleHttpClient:
<?php
Namespace Drupal_module_Controller;
use DrupalCoreControllerBase;
use SymfonyComponentHttpFoundationJsonResponse;
use GuzzleHttpClient;
class GoogleApiIntegrationController extends ControllerBase {
public function getContent() {
// Configure the Guzzle client
$client = new Client([
'base_uri' => 'https://www.googleapis.com/youtube/v3/', // Google API base URL
]);
// Query parameters, including the API key
$params = [
'query' => [
'part' => 'snippet',
'q' => 'cats', // Example query - search for cat videos
'key' => 'YOUR_API_KEY', // Replace 'YOUR_API_KEY' with your own Google API key
],
];
// Perform a GET request
$response = $client->get('search', $params);
// Decode the response JSON
$data = json_decode($response->getBody());
// Process data from the response
$results = [];
foreach ($data->items as $item) {
// You can process video data here, such as displaying titles and descriptions
$results[] = [
'title' => $item->snippet->title,
'description' => $item->snippet->description,
];
}
// Return the results as a JSON response
return new JsonResponse($results);
}
}
Drupal integration through modules
Connecting Drupal with other systems via modules is a popular approach to extend the website's functionality. Especially if it's third-party systems that integrate with our web page.
Examples of popular modules include:
- RESTful Web Services: the module described above allows us to create REST resources and handle HTTP requests, which works well for communicating with other applications and services.
- Feeds: the Feeds module enables us to import and export data from different sources, which is helpful for synchronizing content with external systems. For example, with the Feeds module, we can define the data source and field mapping between Drupal and another system.
- Create Views: the Views module allows us to create custom views that use data from other systems and display them on our Drupal website. Views can also be used to present data from other systems. Particularly useful in integrations is also the option to expose data in XML, CSV, or other formats, which external systems can easily consume. We can use the Views data export module for this purpose.
Drupal integration by this method is a practical solution for various types of projects. There are dedicated modules for specific systems that are ready to use or significantly speed up integration. A few examples are:
- Jira – a project management tool,
- PayPal – an online payment system,
- HubSpot – a CRM platform,
- MailChimp – a marketing automation and e-mail marketing platform.
More such components can be found on Drupal.org in the Third-party Integration
category.
Drupal Salesforce integration
Salesforce is one of the largest and best-known customer relationship management software companies. It offers various CRM (Customer Relationship Management) solutions and tools for automating business processes, marketing, customer service, and data analysis.
Using its example, we’ll show how easy it is to integrate this kind of system with Drupal.
First, we can use a module available on Drupal.org – Salesforce.
The main component contains a set of modules that support integration with Salesforce by synchronizing various Entities (e.g., users, nodes, files) with Salesforce objects (e.g., contacts, leads). It supports uploading data from Drupal to Salesforce, as well as downloading or importing data from Salesforce to Drupal. Changes can be made in real-time or asynchronously in batches when running cron.
If, for some reason, we need more custom solutions, we can extend this with our own module. Salesforce API has classes and a set of hooks for extension, such as:
/**
* Implement an EventSubscriber on SalesforceEvents::PUSH_PARAMS.
*/
function hook_salesforce_push_params_alter() {
}
/**
* Implement an EventSubscriber on SalesforceEvents::PUSH_SUCCESS.
*/
function hook_salesforce_push_success() {
}
/**
* Implement an EventSubscriber on SalesforceEvents::PUSH_FAIL.
*/
function hook_salesforce_push_fail() {
}
We can also use a dedicated SDK for PHP, downloaded with Composer, which allows us to easily receive and send data to Salesforce.
Drupal integrations through Zapier
System integration through Zapier is the process of connecting different applications, tools, and systems to automate tasks and transfer data between them using the platform above. Zapier enables the creation of rules or "zaps" that determine what will happen when a certain event occurs in one system and what actions will be taken in another.
Why is integration with Zapier useful, and what benefits does it bring?
- Simple and fast connection: Integration with systems using Zapier is easy to set up and doesn’t require advanced technical knowledge. We can create our own zaps, customizing them to suit our needs in minutes.
- Task automation: Zapier enables the automation of many tasks and processes, saving time and avoiding human error. This allows us to focus on more strategic aspects of the business.
- Expanded functionality: With support for hundreds of applications and tools, Zapier allows us to create more advanced and integrated solutions. We can synchronize data, generate notifications, create reports, and more, combining different systems into a cohesive work environment.
An example of a simple Drupal integration with Excel via Zapier:
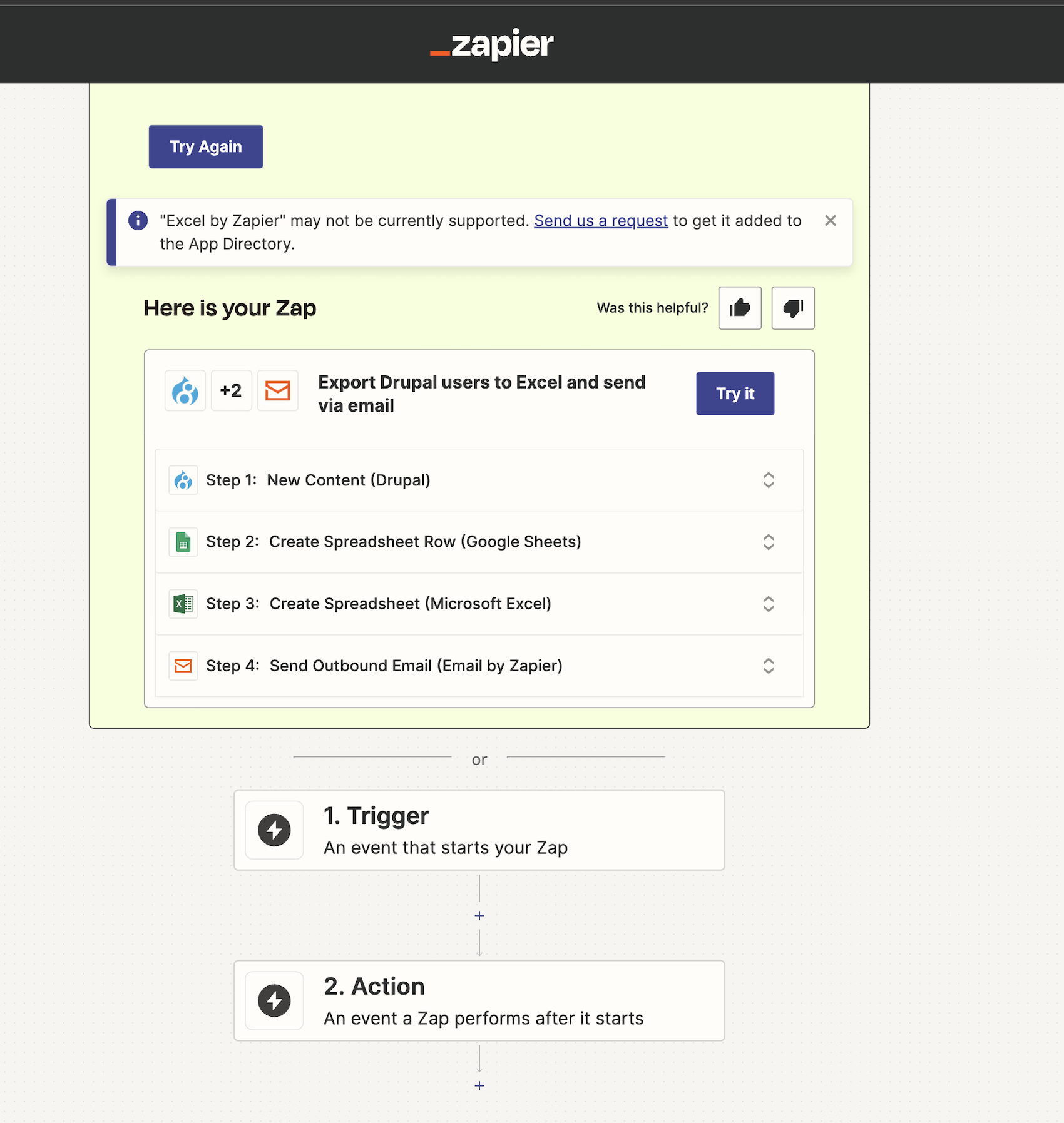
Source: Zapier
Drupal integrations - summary
Drupal is a highly flexible system in terms of extensibility, offering unlimited integration with other solutions and tools. As an open source software, it has a wide range of integration modules available on Drupal.org, which allow us to connect a website to various applications, including CRM, ecommerce, social media, etc.
With its own API and Symfony components, Drupal enables us to create custom integrations, giving us complete control over the communication process between systems. Protecting the data is a priority, and Drupal maintains the highest security standards during integration. If you need help with this tool for creating personalized and integrated websites, get support from an experienced Drupal agency.