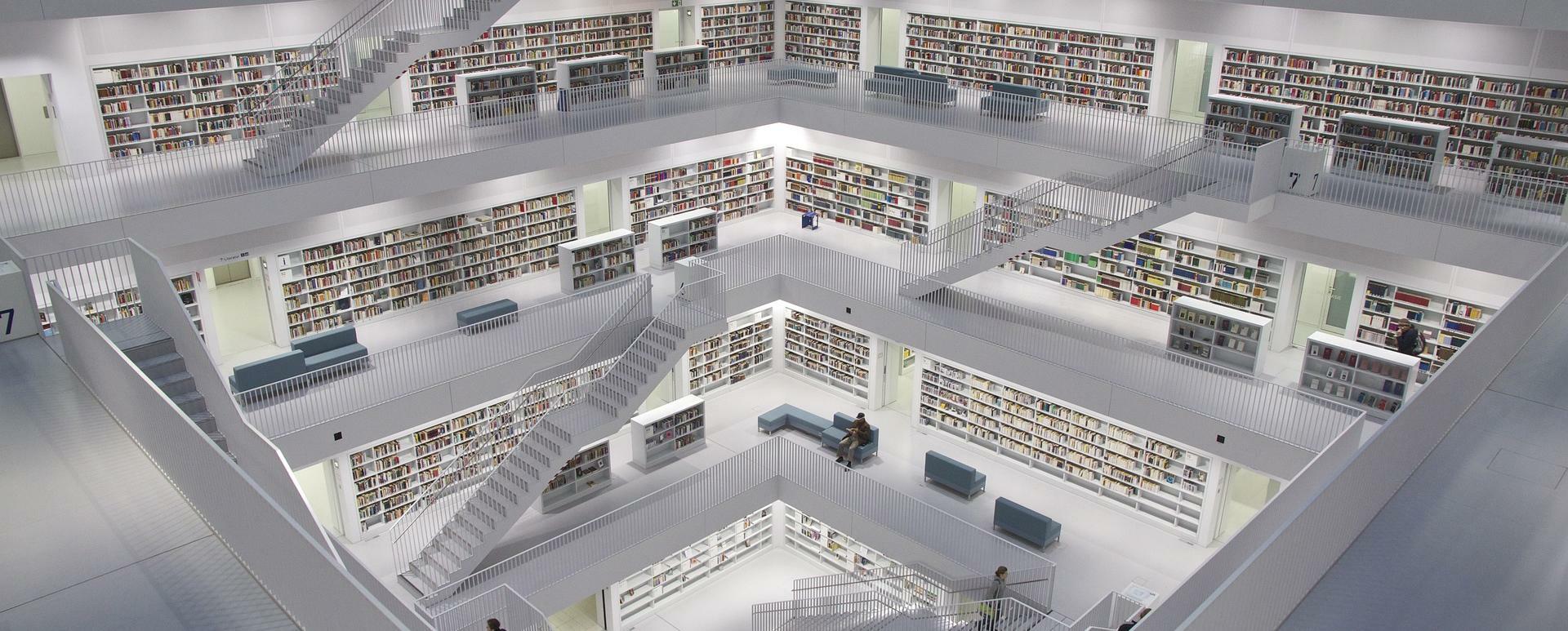
Composer in PHP
Managing libraries, their dependencies and versions is a problem that many technologies and programming languages have encountered in their history. The PHP world has also suffered from difficulties in managing and distributing libraries in the past. Check out how to deal with package management in a PHP application using the Composer dependency manager now.
Until PHP lacked a dependency manager, developers often dealt with having to "reinvent the wheel". Instead of using the existing solutions, they decided to implement some functionalities on their own. In other cases, the software being used was often not updated (packages became insecure) or collecting all dependent libraries simply generated a lot of difficulties and took a long time.
After years of apathy in this field, also for PHP, it was decided to develop an application that allows to freely manage it – following in the footsteps of solutions such as bundler for the ruby language, or npm for node.js, or many others.
The answer to these problems was Composer – a package manager, well known among the PHP developers' community, and one which I am going to present in this article, especially to those who have not had the opportunity to work with it yet.
What is Composer
In the simplest words, Composer is nothing more than an application launched from the command line (the so-called CLI – Command Line Interface), written in PHP. It is designed to manage libraries and scripts for that language. The first works on its creation began in 2011, and after less than a year they were completed with the release of the first official alpha1 version (03.2012). The current stable version is 2.0.7, released in November 2020.
When to use it
Taking into account my own experience working with Drupal developers at Droptica and PHP programmers, I know that there is only one case when it really does not make sense to use Composer – when you do not have a single dependency on any library.
Of course, nowadays, it is hard to imagine a project that would not benefit in any way from the achievements of other programmers and implemented a particular functionality from scratch. Due to the above, and because of the ease of use and installation, Composer is worth using in any situation. It provides an easy way to download, update or terminate dependencies for the library needed for your project.
How to install
Installing Composer is relatively simple, provided that you have PHP 5.3.2 installed in your system (or in a suitable container if you, e.g. use solutions like Docker). Of course, the process itself varies depending on the operating system you are using. The detailed installation process can be found on the Composer official website.
For the Windows system the simplest solution is to download and launch the Composer-Setup.exe file, and then go through the whole installation process. In turn, for the Linux/Unix/macOS systems, installation is being carried out in several steps. First, you download and verify the .phar file (PHP Archive):
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');"
php -r "if (hash_file('sha384', 'composer-setup.php') === '756890a4488ce9024fc62c56153228907f1545c228516cbf63f885e036d37e9a59d27d63f46af1d4d07ee0f76181c7d3') { echo 'Installer verified'; } else { echo 'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;"
Keep in mind that the hash being compared varies with each version released, so always compare it with the value published on the official webpage with checksums.
Then you run the installation, in the basic version – locally
php composer-setup.php
or for global use.
php composer-setup.php --install-dir=/usr/local/bin --filename=composer
In the last step, you delete the unnecessary installation file.
php -r "unlink('composer-setup.php');"
Great, now you can fully enjoy the abilities of Composer in your system.
How to use
Composer, like many applications of this type, offers a number of functionalities that it would not make sense to mention in this article (more detailed information can be found in the official documentation). However, I would like to present here some commands that are most useful in the developer's everyday work.
Project initialisation
composer init
With this command, you initialise a new project using Composer. After its execution, you create the composer.json file, in which all your project data is being stored, such as the project name, details about the author and – most importantly – all libraries used by them.
Installation of an existing project
If you already have an existing project (e.g. you cloned some existing repository), usually one command is enough to install all the same packages that someone published for their application (the list of installed versions of packages is being stored in the composer.lock file).
composer install
Adding, removing, and updating a library
The scheme of all these commands remains the same. Basically, only the keyword for a given operation changes:
- require - for adding,
- remove - for removing,
- update - for updating a package.
For example, in order to add a new library as a dependency to your project (in the background, the dependencies for the library you are downloading are also being terminated), just use the require command:
composer require <vendor name>/<project name>
an example of a command to update the Drupal core would look like this:
composer update drupal/core
For example: let us assume a scenario in which you create an application to generate invoices in the form of .pdf files, which are then being sent to an e-mail address. Of course, you do not want to write everything from scratch but add the libraries you need in a simple manner, so you will use Composer. First, you will complete a PDF library, e.g. dompdf:
composer require dompdf/dompdf
Done! The above command will install the latest version of this library to your project, without any additional interaction on your part. Now it is time for handling the sending of e-mail messages. You will also use a ready-made solution here – PHPMailer. Before you do that, let us assume that for some reason you had a bad experience working with this library in the latest version – 6, but you have worked a lot with version 5. It poses no problem with Composer – you add what you need, defining a specific major version:
composer require phpmailer/phpmailer:"^5"
The Composer's syntax allows for various designations of the necessary versions. These can also be minor versions – you can point to a specific branch, e.g. dev-develop, to branch develop in a given library. You can also indicate a specific version, e.g. 5.2.28, in order to download exactly the one, e.g. because it is a well-tested and working version.
After executing the above commands, a vendor directory will be created, containing the downloaded libraries along with their dependencies:
The composer.json file will also be updated accordingly, and the composer.lock file will be generated. The final structure in the project directory is as follows:
As you can see, having a set of several commands, you can basically successfully start working on any project – in most cases, you will not need anything else, and all the magic is done for you in the background.
What do you get
Composer is primarily a comprehensive tool commonly used in PHP projects, while it is also written in PHP. This is a huge advantage because any potential developer joining your team or the company you intend to work with surely came across it and used it before. The simple installer and running basic commands downright encourage you to use it from the very beginning when working on any project.
In addition, the ease with which you can install any PHP library while having an influence on its version or update options allows for significant savings in developers' time and available resources. Thanks to packages like sensiolabs/security-checker you can also independently check the available security updates, increasing the security of your own application. It is typical for our daily work; especially when, e.g. we provide continuous monitoring of Drupal-based websites the ability to quickly update using Composer is indispensable, and the process itself is fast and efficient.
Summary
As an experienced developer, I cannot imagine my daily work without the use of Composer. The tools like dependency manager or package manager are common in many technologies, including PHP. The multitude of advantages, such as saving time, ease of use, and simple control over dependencies, make me more effective in my work. If you also want to focus only on the development of your own application code, and at the same time – keep up with the commonly-used standards, then simply use Composer. It is dead easy.