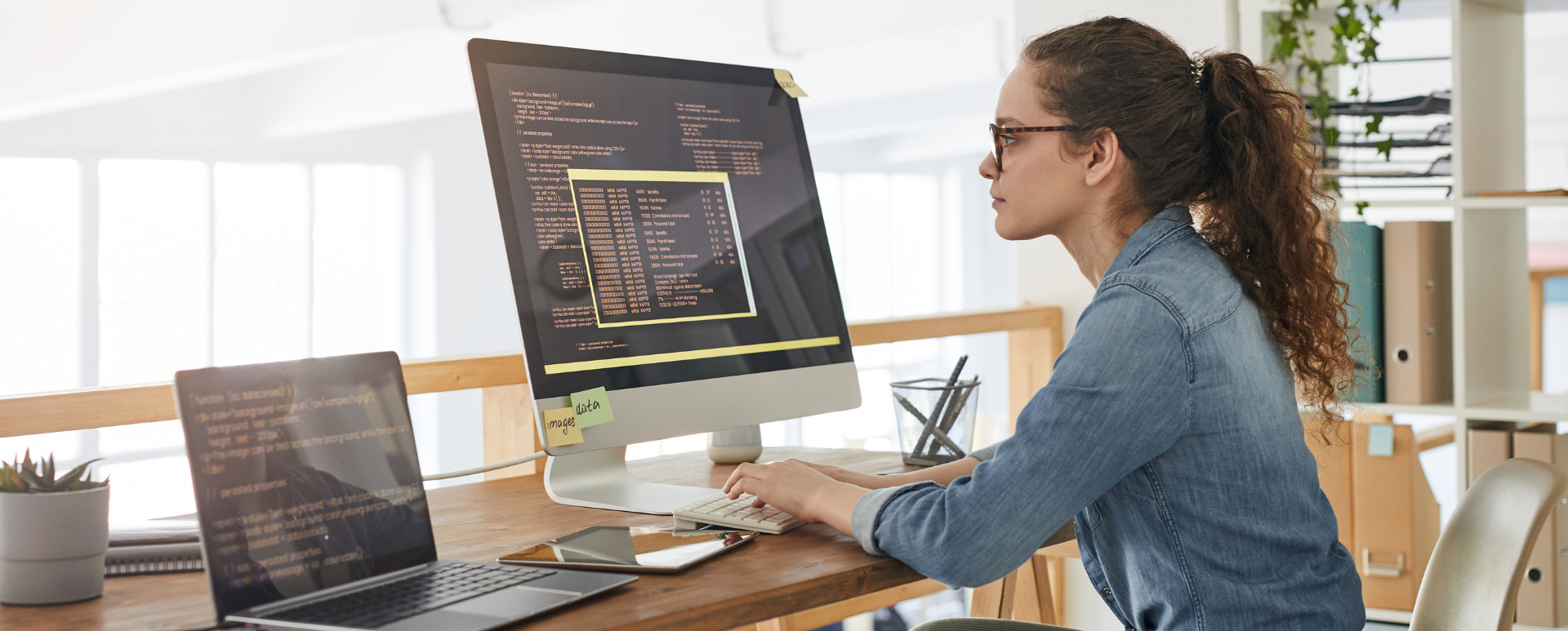
What are Drupal Coding Standards, and How to Use Them in Your Daily Work?
Standardization has a positive effect on any business. There are certain coding standards in the software industry that are needed for successful software development. Coding standards are a set of rules and good practices that make code more legible and easier to develop. In this article, we’ll present what standards are used to create Drupal projects. We will show you the basic aspects that are worth paying attention to and the tools that make work easier.
Coding standards in Drupal
The Drupal community defines a set of coding standards and best practices that should be followed when writing code. They are partly based on the PEAR coding standards. The community's newly proposed coding standards are first discussed in the Coding Standards project.
The coding standards in Drupal are independent of the version of Drupal in which we’re currently working. All new code should follow the current rules. We can find full information on the coding standards in Drupal on the official Drupal website.
Array
Arrays should be formatted using short syntax with spaces separating every element after a comma. We should add spaces before and after the assignment operator “=>”.
$some_array = ['hello', 'world', 'foo' => 'bar'];
If the array declaration exceeds 80 characters, we should break it down into several lines.
$form['title'] = [
'#type' => 'textfield',
'#title' => t('Title'),
'#size' => 60,
'#maxlength' => 128,
'#description' => t('The title of your node.'),
];
Note the comma in the last line – this isn't an error. This way, we're able to prevent errors when adding new array elements in the future.
Warning! The short syntax is available in PHP from version 5.4. In older versions, we should use long syntax.
If else
The if else structure looks like in the example below. Note the arrangement of parentheses, spaces, and curly brackets. Control statements should have one space between the condition and the opening parenthesis.
if (condition1 || condition2) {
action1;
}
elseif (condition3 && condition4) {
action2;
}
else {
defaultaction;
}
Warning! Never use else if. You may use elseif instead.
Switch
We should always use round parentheses in control statements, even when they're technically optional, like in the switch control statement. This makes code more legible.
switch (condition) {
case 1:
action1;
break;
case 2:
action2;
break;
default:
defaultaction;
}
Do while
Below we show an example of the do while control statement. We add a space between the do and the opening parenthesis. The condition must be in the same line as the statement's closing parenthesis, separated from it by a space.
do {
actions;
} while ($condition);
Code line length
Each line of code shouldn't be longer than 80 characters. The exception may be a function, class, or variable declaration with a very long name.
Breaking down conditions
Conditions shouldn't exceed a single line. Drupal encourages us to take the value of a condition into a variable for legibility. Below is an example of how to rework too long condition lines.
Wrong:
if ((isset($key) && !empty($user->uid) && $key == $user->uid) || (isset($user->cache) ? $user->cache : '') == ip_address() || isset($value) && $value >= time())) {
...
}
Right:
$is_valid_user = isset($key) && !empty($user->uid) && $key == $user->uid;
// IP must match the cache to prevent session spoofing.
$is_valid_cache = isset($user->cache) ? $user->cache == ip_address() : FALSE;
// Alternatively, if the request query parameter is in the future, then it
// is always valid, because the galaxy will implode and collapse anyway.
$is_valid_query = $is_valid_cache || (isset($value) && $value >= time());
if ($is_valid_user || $is_valid_query) {
...
}
Example function
function funstuff_system($field) {
$system["description"] = t("This module inserts funny text into posts randomly.");
return $system[$field];
}
Arguments with default values must always be at the end. Anonymous functions, on the other hand, should have a space between the "function" and its parameters, like in the following example:
array_map(function ($item) use ($id) {
return $item[$id];
}, $items);
Drupal JavaScript coding standards
Always add your JS scripts using Drupal.behavior. Function names should start with the name of the module or theme that declares the function in order to avoid name collisions.
Drupal.behaviors.tableDrag = function (context) {
Object.keys(Drupal.settings.tableDrag).forEach(function (base) {
$('#' + base).once('tabledrag', addBehavior);
});
};
Drupal CSS coding standards
Below, there are some rules that'll help you with correct CSS coding.
Avoid relying on the HTML structure
CSS should define the look of an element in any place, and in the place where it appears. Never use HTML sectors.
BEM
A good practice when writing styles in Drupal is to follow the BEM methodology. Writing CSS code in this way prevents multiple nesting and reliance on markup structure and overly generic class names. The methodology breaks down all elements into three categories:
- Blocks – they are usually single components, for example, navigation. This looks like a single class in code, e.g. .navigation.
- Elements – that is, subordinate parts of a block, such as a link in the navigation. The element name should be separated from the block name by two underscores, e.g. .navigation__link, .navigation__item.
- Modifiers – specific variants of blocks and elements. The modifier's name should be separated from the base by two dashes, for example: .navigation–dark, .navigation__link–active.
Drupal Twig coding standards
All coding standards for Twig, the template engine for PHP, are described on the official Twig documentation page.
Expressions
Expressions in Twig are very similar to expressions in PHP. They're most often used to check if a variable exists, to declare loops and new variables in templates.
Checking if a variable is available for printing:
{% if foo %}
<div>{{ foo }}</div>
{% endif %}
Loop:
{% for key, value in items %}
<div class="{{ key }}">{{ value }}</div>
{% endfor %}
New variables declaration:
{% set list = ['Alice', 'Bob'] %}
{% set text = ':person is a Twig fan'|t({':person': list[0] }) %}
Filters
Some of Drupal's most popular features, such as t and url, have been made available as filters in Twig templates. They are triggered with the pipe character | .
<div class="preview-text-wrapper">
{{ 'Original'|t }}
</div>
How to use Drupal coding standards on a daily basis?
There are tools available to help you format your code to conform to the standards. Here are some of the tools we use during Drupal development.
- Code Sniffer – a tool to help you write code for Drupal or its modules. It's able to detect and automatically fix standard coding and formatting errors.
- Coder Drupal module – when integrated with Code Sniffer, it helps to fix bugs in the coding standard.
- CSSComb - a tool for sorting and formatting CSS code.
When using tools integrated with the IDE, the developer doesn't need to know all the coding standards by heart and can be sure that there'll be no unnecessary spaces or wrong tabulators left in the code. They'll also find out if any changes are introduced to the coding convention.
In conclusion, it’s very important that the development team adhere to the appropriate coding conventions and standards that'll guide them during software development. This will help maintain the code's quality and reduce the time new developers spend trying to understand a complex codebase.