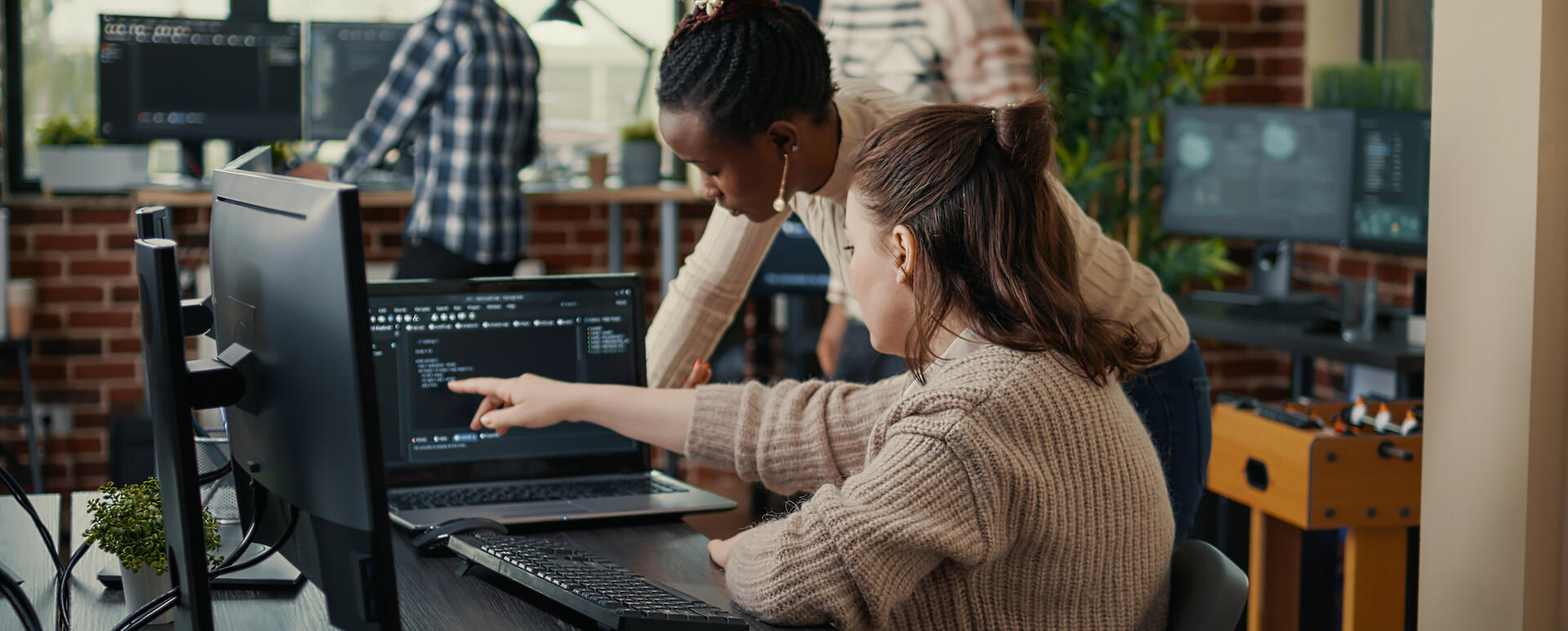
What is TypeScript and Why Will it Work for Your Projects?
TypeScript is an innovative programming language that is gaining popularity among developers. It extends the well-known JavaScript with the ability to type variables, which brings numerous benefits (such as easier error detection in the code). In this article, we’ll present TypeScript’s genesis, functionalities, advantages, and differences from JS. We’ll also exemplify this language application in projects and tools to make working with it easier.
What is TypeScript?
TypeScript is a programming language created by Microsoft in 2012. Its creator is Anders Hejlsberg, also known as the C# language father. TypeScript is a superset of JavaScript, meaning any valid JS code is also a valid TS code. In practice, TypeScript extends JavaScript's capabilities with optional static typing, new data structures such as Enums and Classes, and other features mentioned later in the article. TS also has an active developers community creating libraries that simplify developers' work by adding typing to existing JS projects. As a result, they are adapted to work with TypeScript.
The main TypeScript creation goal was to introduce a stronger type system to JavaScript, which allows top-down types determination of developer-defined variables to facilitate building large, scalable applications. The language has become popular among developers because of its flexibility, the possibilities offered by static typing, and the ease of integration with existing JS projects.
TypeScript differs from JavaScript mainly in the abovementioned system, which allows for better management and code control. In addition, TypeScript introduces elements such as interfaces, abstract classes, and decorators, which makes the code more transparent and easier to maintain.
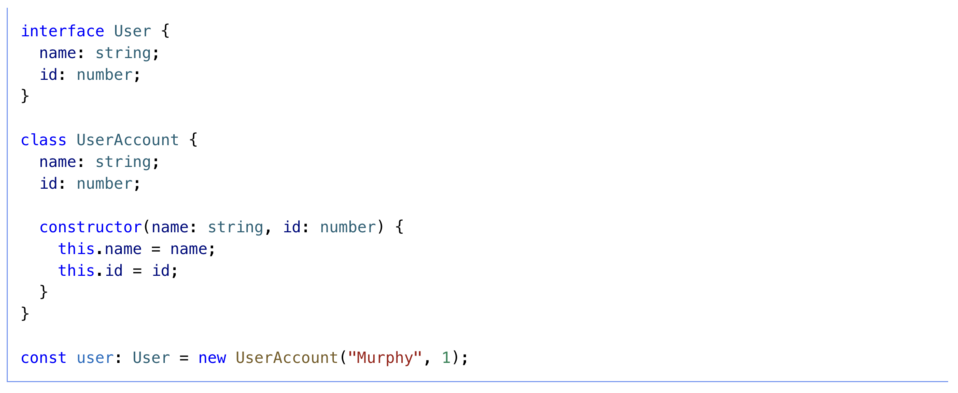
Source: TypeScript
What are the TypeScript features?
TypeScript offers many useful features to streamline the process of developing, testing, and maintaining applications. Proper language use will make it virtually impossible to provide users with code in which we refer to non-existent or erroneous variables.
The most important TypeScript features are:
- Static typing: TypeScript allows you to define data types for variables, functions, and objects, which helps detect errors at the compilation stage. This enables developers to identify and fix potential problems faster.
- Interfaces: implementing interfaces in TypeScript is a way to define the objects' structure. They allow you to define the expected data structure, making it easier to create clear contracts between different application parts. This way, when sending or receiving data on a particular interface, we know exactly what values to expect.
- Classes and Inheritance: TypeScript introduces classes and inheritance, familiar from languages such as Java and C#. They let us create a hierarchy of objects and easily manage related functions and properties. TypeScript doesn’t support multi-base inheritance, which means we can use only one base class.
- Decorators: these particular constructs allow you to modify or extend classes, methods, or properties of objects. They facilitate the creation of clean, easily extensible code. Decorators extend the behavior of a class, field, method, and parameter at runtime, which is how they differ from the inheritance taking place when the code is compiled.
- Modules: TypeScript is built using separate modules that make it easy to create multiple independent application parts without importing unused components. In the latest TS version, rewriting components to a modular approach allows for better source code management, resulting in better organization and team collaboration. Their classes, variables, or functions are only visible within the scope of a module.
- Integration with development tools: the TS language integrates well with popular tools for developers, such as Visual Studio Code, WebStorm, and Linters. It simplifies the application development process and provides support for different working environments.
What is TypeScript used for?
TypeScript is used in many types of projects, both frontend and backend. With its advanced features, it allows you to create web applications, games, libraries, frameworks, and developer tools. The language is particularly well suited for large and complex projects, where the type system and other TypeScript features help organize and maintain code.
One of the popular TypeScript uses is to develop applications based on frontend frameworks such as Angular, React, or Vue. TypeScript enables better control over data types and component behavior, resulting in more reliable applications.
TypeScript can also be used to develop server-side applications based on the Node.js platform, allowing the same language to be employed on the frontend and backend.
A simple interface example that we could use in an actual project:
interface User {
id: number;
name: string;
surname: string;
email: string;
active: boolean;
}
In the given example, we’re defining a user interface that we’ll be able to use on both application sides (in the frontend and backend), which will allow us to avoid mistakes such as misassigning variables or referring to non-existent ones. The latter refers to the situation when we would mistakenly try to change the user’s name with a typo in the variable name, TypeScript would draw our attention to it right away. The interface will also serve us in a situation where we want to display all available data about a user. This is especially useful for interfaces with more fields.
Why use TypeScript?
TypeScript provides many benefits that translate into better code quality, increased productivity for experienced developers, and faster workflow. Using TS in projects allows you to take advantage of its advanced features and flexibility, which is why it’s becoming an increasingly popular language among developers.
Here are some of the main TypeScript advantages:
- Immediate typing errors detection: with static typing, the TypeScript-enabled development environment will detect typing or variable naming errors on the fly and bring them to our attention.
- Better code readability: TypeScript introduces numerous features, such as interfaces, classes, and decorators, that make it easier to organize code and make it more transparent to other developers.
- Easier refactoring: static typing and support for developer tools make TypeScript easier to refactor code, which is especially important for large and complex projects.
- Integration with existing JavaScript projects: TypeScript is fully compatible with JavaScript, allowing TS to be gradually implemented in existing projects and to work with JS-based libraries and tools.
- Support for modern language features: TS allows you to use the latest ECMAScript features even in older browsers, thanks to transpiration to JavaScript's older version. This gives you the ability to use new language features without worrying about their compatibility on the HTML client side.
- Increased productivity: TypeScript enables writing code faster and more efficiently, thanks to better support for autocomplete, code navigation, and smart suggestions that popular developer tools offer.
Additional tools for developers
When working with TypeScript, it’s a good idea to use additional developer tools to make it easier to write code, ensure its quality and optimize the testing process.
Here are the five most popular tools used in TypeScript projects:
- Visual Studio Code: it’s a popular development environment (IDE) created by Microsoft that offers excellent support for TypeScript. Visual Studio Code's advantages include an intuitive user interface, rich configuration capabilities, a vast extension library, and integration with version control systems. The disadvantage may be higher resource consumption compared to lighter text editors.
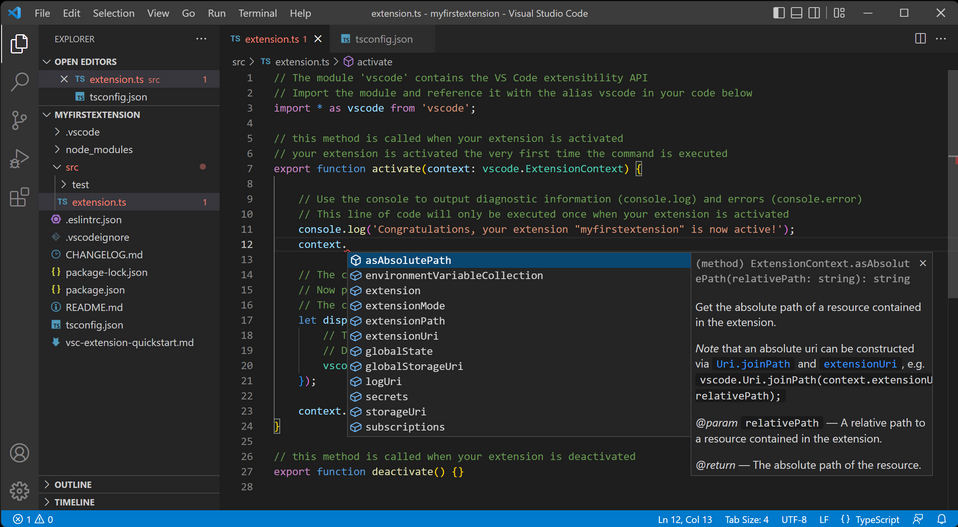
Source: Visual Studio Code
- TSLint: it’s a static analysis tool for TypeScript code that helps maintain code quality by detecting potential errors and non-compliance with standards. The TSLint’s advantages are its configurability and ability to integrate with other tools such as Webpack and Gulp. The disadvantage is the gradual phasing out of TSLint, which was created strictly to support TypeScript, in favor of ESLint (which, in turn, initially supported only JavaScript, but was extended to include TypeScript functionality).
- Webpack: it’s an application-building tool that allows you to combine, optimize and minify TypeScript, JavaScript, CSS files, and other resources. Webpack's advantages include flexibility, the ability to load modules, integration with other tools, and support for hot module replacement (this feature allows you to update only the part of the code that you have changed on an already compiled page, speeding up the development and testing of changes). The disadvantage is the relatively high entry threshold for novice developers.
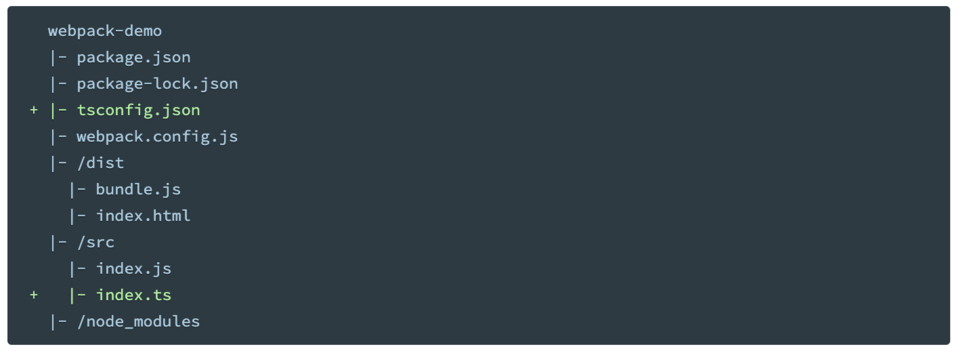
Source: Webpack.js
- TypeORM: it’s an Object Relational Mapper (ORM) tool for TypeScript for object-relational mapping. It facilitates working with databases in Node.js-based applications. TypeORM’s advantages include support for multiple database systems, the use of decorators, and integration with TypeScript's type system. Disadvantages may include slightly higher complexity than other ORMs and lower performance for some queries.
- Jest: it’s a popular library for testing TypeScript and JavaScript code, developed by Facebook. Jest's advantages include speed, ease of configuration, support for snapshot testing (comparing a newly generated part of the application with previous snapshots to avoid unwanted changes), and the ability to run tests simultaneously. A disadvantage may be the slightly lower flexibility (manifested in marking the aforementioned snapshot changes as unwanted despite their correctness) compared to other testing frameworks such as Mocha or Jasmine.
What is TypeScript - summary
TypeScript is a programming language developed by Microsoft that extends the capabilities of JavaScript with optional static typing and many other features. It’ll allow you to scale small projects and develop large ones, and advanced TypeScript features (e.g., interfaces, modularity, and facilitated refactoring) will help you organize and maintain your code.
Supported by a wide range of development tools, TypeScript is becoming increasingly popular among developers who are looking for the flexibility, performance, and type reliability that the language offers. Developers at Droptica are also eager to use it for projects involving, for example, frontend development and can help you if you need support in building or developing web applications.